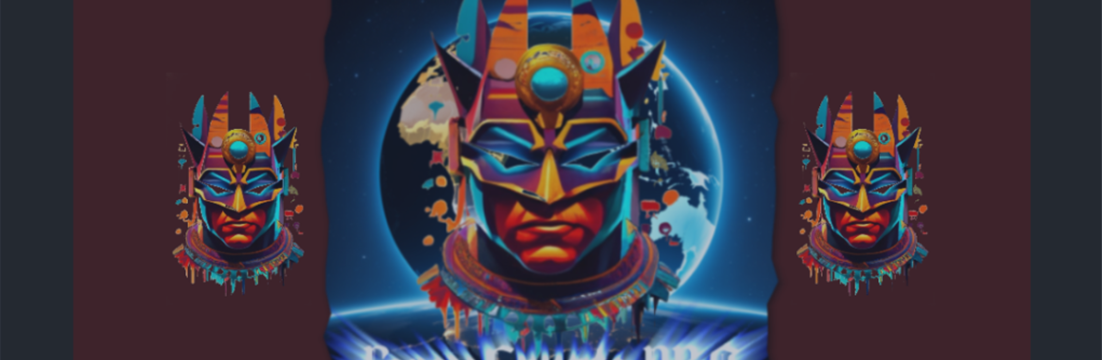
/*
Guidelines:
Purchase when RSI Oversold (<45) and EMA(5) crosses above EMA(10)
Promote when RSI Overbought (>55) and EMA(5) crosses under EMA(10)
**added
Purchase Cease loss at nearest swing low(5 fractal)
Purchase TakeProfit at 1:1
Promote Cease loss at nearest swing excessive(5 fractal)
Promote TakeProfit at 1:1
#property copyright “© 2024, FOREXinstan.com & Co. All rights reserved”
#property model “21.4”
#outline Pref “EA-RSI”
#property description “Superior software program is designed particularly for skilled merchants and futures corporations.”
#property description “It permits customers to investigate methods concurrently on eight lively pairs, together with shadows.”
#property description ” “
#property description “SUPPORT 24/7: Scalping technique from reversal code in telegram bot: T.me/FOREXnowTV”
#property strict
#outline app_name “ChatGPT – RSI MA Cross”
#outline app_magic 112233
#embrace <incRSI-MA.mqh>
#embrace <MovingAverages.mqh>
//
// Quick MA
enter int InpFastMAPeriod = 5; // Quick MA Bars
enter ENUM_MA_METHOD InpFastMAMethod = MODE_EMA; // Quick MA Technique
enter ENUM_APPLIED_PRICE InpFastMAAppliedPrice = PRICE_CLOSE; // Quick MA Utilized Worth
// Gradual MA
enter int InpSlowMAPeriod = 10; // Gradual MA Bars
enter ENUM_MA_METHOD InpSlowMAMethod = MODE_EMA; // Gradual MA Technique
enter ENUM_APPLIED_PRICE InpSlowMAAppliedPrice = PRICE_CLOSE; // Gradual MA Utilized Worth
enter int InpRSIPeriod = 50; // RSI Interval
enter ENUM_APPLIED_PRICE InpRSIAppliedPrice = PRICE_CLOSE; // RSI Utilized Worth
enter double InpRSIOverboughtLevel = 80; // RSI Overbought
enter double InpRSIOversoldLevel = 15; // RSI Oversold
enter int InpSwingPeriod = 5; // Swing excessive/low interval
enter double InpTakeProfitRatio = 1.0; // tp:sl ratio
// Primary inputs
enter double InpOrderSize = 0.01; // Order measurement in heaps
enter int InpMagic = app_magic; // Magic quantity
enter string InpTradeComment = app_name; // Commerce remark
// buffers for the shifting averages
double BufferFastMA[];
double BufferSlowMA[];
double BufferRSI[];
const int BufferValuesRequired = 3;
// Initialisation
int OnInit() {
// Reset the brand new bar
IsNewBar();
// MT4 Particular
ArrayResize( BufferFastMA, BufferValuesRequired );
ArrayResize( BufferSlowMA, BufferValuesRequired );
ArrayResize( BufferRSI , BufferValuesRequired );
// finish MT4 Particular
return ( INIT_SUCCEEDED );
void OnDeinit( const int cause ) {}
void OnTick() {
// Enter a commerce on a cross of quick MA over gradual MA
// Exit any present reverse on the similar time
// means there can solely be one commerce open at a time
// Not an important buying and selling technique however demo
bool newBar = IsNewBar();
bool conditionOpenBuy = false;
bool conditionOpenSell = false;
if ( !newBar ) return;
// Get the quick and gradual ma values for bar 1 and bar 2
if ( !FillBuffers( BufferValuesRequired ) ) return;
// Evaluate, if Quick 1 is above Gradual 1 and Quick 2 will not be above Gradual 2 then
// there’s a cross up
conditionOpenBuy = ( CrossUp ( BufferFastMA, BufferSlowMA, 1 ) && ( BufferRSI[1] < InpRSIOversoldLevel ) );
conditionOpenSell = ( CrossDown( BufferFastMA, BufferSlowMA, 1 ) && ( BufferRSI[1] > InpRSIOverboughtLevel ) );
double stopLossPrice = 0;
if ( conditionOpenBuy ) {
SwingLow( 0, InpSwingPeriod, stopLossPrice );
TradeOpenSLPriceTPRatio( ORDER_TYPE_BUY, InpOrderSize, InpTradeComment, InpMagic, stopLossPrice, InpTakeProfitRatio );
return;
if ( conditionOpenSell ) {
SwingHigh( 0, InpSwingPeriod, stopLossPrice );
TradeOpenSLPriceTPRatio( ORDER_TYPE_SELL, InpOrderSize, InpTradeComment, InpMagic, stopLossPrice, InpTakeProfitRatio );
double PipsToDouble( int pips ) { return PointsToDouble( PipsToPoints( pips ) ); }
int PipsToPoints( int pips ) Digits() == 5 ) ? 10 : 1 );
double PointsToDouble( int factors ) {
return factors * Level(); // simply variety of factors * measurement of some extent
bool IsNewBar() {
static datetime previous_time = 0;
datetime current_time = iTime( Image(), Interval(), 0 );
if ( previous_time != current_time ) {
previous_time = current_time;
return true;
return false;
// MT4 Particular
// Load values from the indications into buffers
bool FillBuffers( int valuesRequired ) {
for ( int i = 0; i < valuesRequired; i++ ) {
BufferFastMA[i] = iMA( Image(), Interval(), InpFastMAPeriod, 0, InpFastMAMethod, InpFastMAAppliedPrice, i );
BufferSlowMA[i] = iMA( Image(), Interval(), InpSlowMAPeriod, 0, InpSlowMAMethod, InpSlowMAAppliedPrice, i );
BufferRSI[i] = iRSI( Image(), Interval(), InpRSIPeriod, InpRSIAppliedPrice, i );
return true;
// Open a market value commerce given a cease loss value and take revenue ratio
void TradeOpenSLPriceTPRatio( ENUM_ORDER_TYPE kind, double quantity, string tradeComment, int magic, double stopLossPrice = 0, double takeProfitRatio = 0 ) {
if ( stopLossPrice < 0 ) return;
double value;
double closePrice;
double sl = stopLossPrice;
double tp = 0;
double stopsLevel = PointsToDouble( ( int )SymbolInfoInteger( Image(), SYMBOL_TRADE_STOPS_LEVEL ) );
RefreshRates();
if ( kind == ORDER_TYPE_BUY ) {
value = SymbolInfoDouble( Image(), SYMBOL_ASK );
closePrice = SymbolInfoDouble( Image(), SYMBOL_BID );
if ( stopLossPrice > ( closePrice – stopsLevel ) ) return;
else {
value = SymbolInfoDouble( Image(), SYMBOL_BID );
closePrice = SymbolInfoDouble( Image(), SYMBOL_ASK );
if ( stopLossPrice < ( closePrice + stopsLevel ) ) return;
if ( takeProfitRatio > 0 ) tp = value + ( ( value – stopLossPrice ) * takeProfitRatio);
value = NormalizeDouble( value, Digits() );
sl = NormalizeDouble( sl, Digits() );
tp = NormalizeDouble( tp, Digits() );
if ( !OrderSend( Image(), kind, quantity, value, 0, sl, tp, tradeComment, magic ) ) {
Print( “Open failed for %s, %s, value=%f, sl=%f, tp=%f”, Image(), EnumToString( kind ), value, sl, tp );
// Finish MT4 Particular
// That is all of us
[Reviewer Required > Goodwill 1 month] “Quantum Leap” 2024| eight Pairs, eight TimeFrames, eight Shadows & Reversal Code ➾ 1 Chart